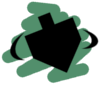 MezzanineEngine
|
Go to the documentation of this file.
40 #ifndef _crossplatformexports_h
41 #define _crossplatformexports_h
106 #define _MEZZ_THREAD_WIN32_
109 #pragma warning( disable : 4251) // Disable the dll import/export warnings on items that are set correctly.
110 #pragma warning( disable : 4244) // Disable the double to float conversions, they are in their by design to minimize floating point rounding during intermediate calculations.
111 #pragma warning( disable : 4127) // Disable conditional expression is constant
112 #pragma warning( disable : 4800) // pugi xml unspecified bool type coercion has performance cost, used only in unit tests
113 #pragma warning( disable : 4221) // interfaces don't generate linkable symbols, well of course they don't
114 #pragma warning( disable : 4512) // Could not generate assignment operator for class that doesn't need one
118 #ifdef EXPORTINGMEZZANINEDLL
119 #define MEZZ_LIB __declspec(dllexport)
121 #define MEZZ_LIB __declspec(dllimport)
122 #endif // \EXPORTINGMEZZANINEDLL
128 #define _MEZZ_THREAD_POSIX_
129 #if defined(__APPLE__) || defined(__MACH__) || defined(__OSX__)
132 #define _MEZZ_THREAD_APPLE_
136 #define _MEZZ_PLATFORM_DEFINED_
140 #ifndef MEZZ_DEPRECATED
141 #if defined(__GNUC__)
142 #define MEZZ_DEPRECATED __attribute__((deprecated))
143 #elif defined(_MSC_VER) && _MSC_VER >= 1300
144 #define MEZZ_DEPRECATED __declspec(deprecated)
146 #define MEZZ_DEPRECATED
155 #ifndef MEZZ_USEBARRIERSEACHFRAME
156 #define MEZZ_USEBARRIERSEACHFRAME
158 #ifndef _MEZZ_MINTHREADS_
159 #undef MEZZ_USEBARRIERSEACHFRAME
172 #ifndef MEZZ_USEATOMICSTODECACHECOMPLETEWORK
173 #define MEZZ_USEATOMICSTODECACHECOMPLETEWORK
175 #ifndef _MEZZ_DECACHEWORKUNIT_
176 #undef MEZZ_USEATOMICSTODECACHECOMPLETEWORK
182 #ifndef MEZZ_FRAMESTOTRACK
183 #define MEZZ_FRAMESTOTRACK 10
185 #ifdef _MEZZ_FRAMESTOTRACK_
186 #undef MEZZ_FRAMESTOTRACK
187 #define MEZZ_FRAMESTOTRACK _MEZZ_FRAMESTOTRACK_
193 #define WINAPI __stdcall
195 #define WINAPI ErrorThisOnlyGoesInwin32Code