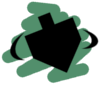 MezzanineEngine
|
40 #ifndef _uidropdownlist_cpp
41 #define _uidropdownlist_cpp
43 #include "UI/uimanager.h"
44 #include "UI/dropdownlist.h"
45 #include "UI/listbox.h"
46 #include "UI/button.h"
47 #include "UI/scrollbar.h"
48 #include "UI/screen.h"
49 #include "Input/inputmanager.h"
50 #include "Input/mouse.h"