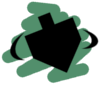 MezzanineEngine
|
47 #include "Audio/audioenumerations.h"
48 #include "Audio/decoder.h"
73 virtual bool IsValid()
const = 0;
113 virtual bool Play() = 0;
118 virtual void Pause() = 0;
123 virtual void Stop() = 0;
129 virtual void Loop(
bool ToLoop) = 0;
139 virtual bool Seek(
const Real Seconds,
bool Relative =
false) = 0;